Display submit api
This documentation explains the mechanics of display and submitting consumer store reviews, including store specific meta-data, to Bazaarvoice using the Mobile SDK.
Introduction
Use the Bazaarvoice Mobile SDKs to enable Ratings and Reviews for Stores functionality, such as displaying store reviews and submitting a review and photo of a store. The API is very similar to the Conversations API for product reviews, with the addition that the BVSDK provides additional meta-info for stores, such as geo-location. Contact Bazaarvoice to set up Ratings and Reviews for Stores.
You will need your apiKeyConversationsStores to implement and use Ratings and Reviews for Stores with Mobile SDKs.
Check out the code samples below to get an overview of what functionality you can use with the BVSDK.
Before you start implementing this Mobile SDK module, verify your installation by checking the steps on the Installation page.
Display store reviews
Use the BVStoreReviewsRequest
object to construct parameters and make the API request. Display the results in the response.results
value, which contain an array of BVReview
objects.
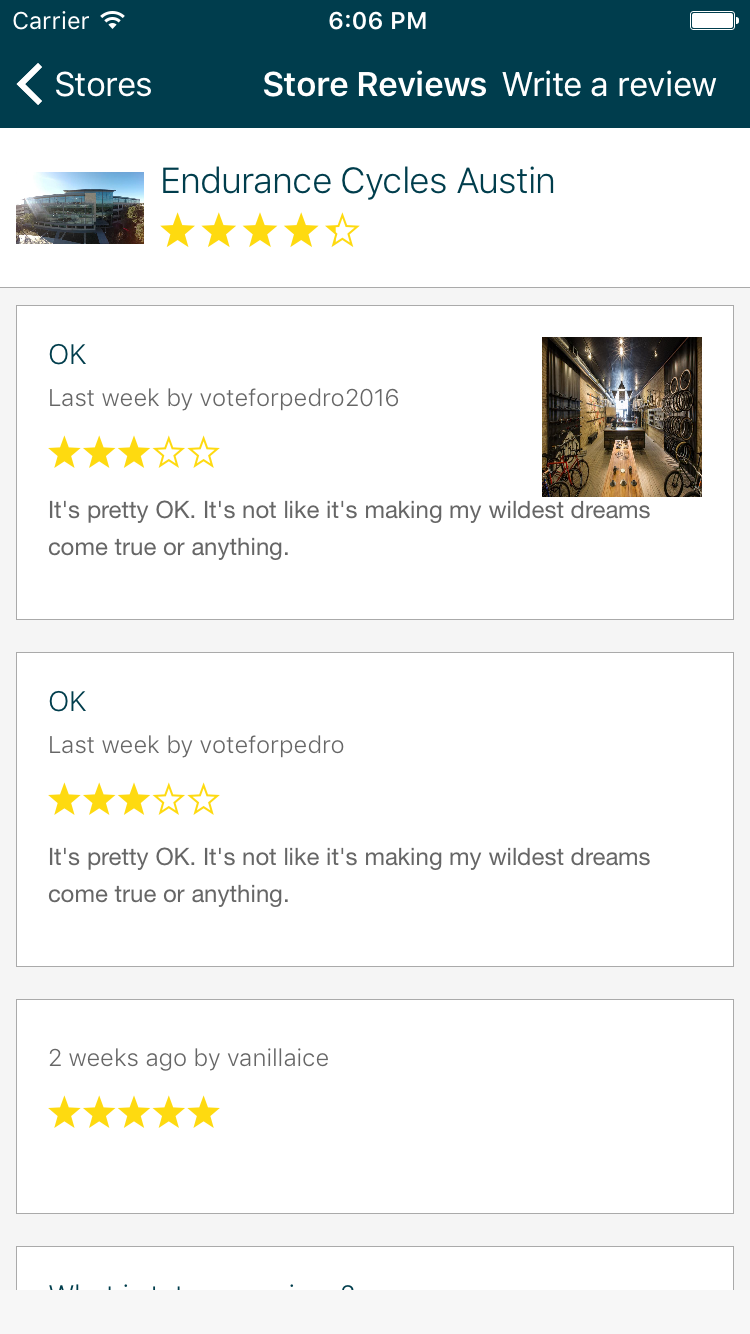
import BVSDK
...
let request = BVStoreReviewsRequest(storeId: store.identifier, limit: 20, offset: Int32(self.reviews.count))
// Sorting and filter FilterOptions
request.addSort(.Rating, order: .Descending)
request.load({ (response) in
// success - show your reviews!
// response.results contains an array of BVReview objects
}) { (error) in
// failure - tell the user something went wrong : (
}
BVStoreReviewsRequest *request = [[BVStoreReviewsRequest alloc] initWithStoreId:@"1" limit:20 offset:0];
[request addSort:BVSortOptionProductsRating order:BVSortOrderDescending];
[request load:^(BVStoreReviewsResponse * _Nonnull response) {
// success
// response.results contains an array of BVReview objects
} failure:^(NSArray * _Nonnull errors) {
// error
// failure - tell the user something went wrong : (
}];
Display store ratings
Use the BVBulkStoreItemsRequest
object to construct parameters and make the API request. Display the results in the response.results
value, which contain an array of BVStore
objects.
The BVBulkStoreItemsRequest
contains two constructors you can use depending whether you want to fetch all the stores with limit and offset, or you just want to fetch one or more stores if you already have the store id.
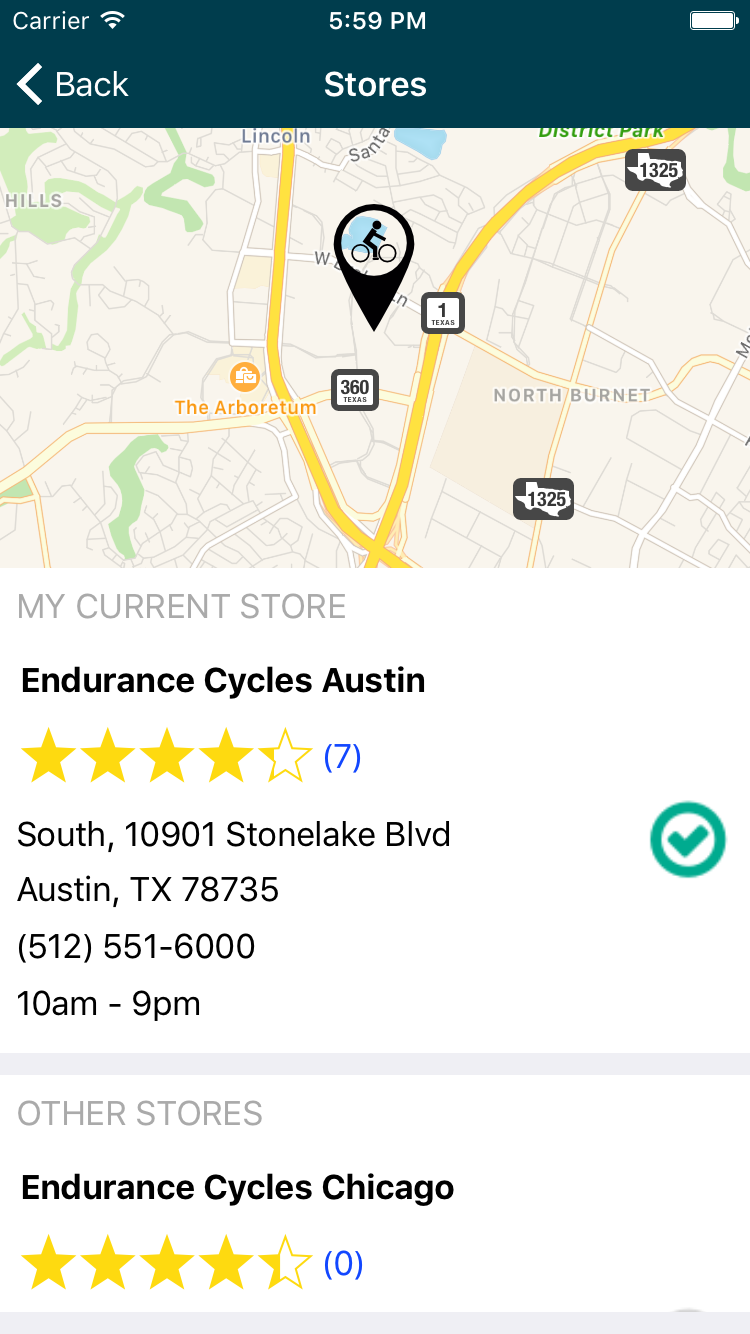
Display stores with limit and offset
import BVSDK
...
let request = BVBulkStoreItemsRequest(20, offset: 0)
request.includeStatistics(.Reviews)
request.load({ (response) in
// success
// Find the array for BVStore objects in response.results
}) { (error) in
// error - tell the user something went wrong : (
}
BVBulkStoreItemsRequest *request = [[BVBulkStoreItemsRequest alloc] init:20 offset:0];
[request load:^(BVBulkStoresResponse * _Nonnull response) {
// Get the BVStore(s) in the response.results array
BVStore* store = response.results.firstObject;
} failure:^(NSArray * _Nonnull errors) {
// handle failure appropriately
}];
Display stores by ID
import BVSDK
...
let req = BVBulkStoreItemsRequest(storeIds: ["1", "2"])
req.load({(response) in
// success
// get the BVStore object(s) in response.results
}){(error) in
// error - tell the user something went wrong : (
}
BVBulkStoreItemsRequest *request = [[BVBulkStoreItemsRequest alloc] initWithStoreIds:@[@"1", @"2"]];
[request load:^(BVBulkStoresResponse * _Nonnull response) {
// Get the BVStore(s) in the response.results array
BVStore* store = response.results.firstObject;
} failure:^(NSArray * _Nonnull errors) {
// handle failure appropriately
}];
Submitting a review on a store
Use the BVStoreReviewSubmission
class to set up your submission form values and submit a review on a single store.
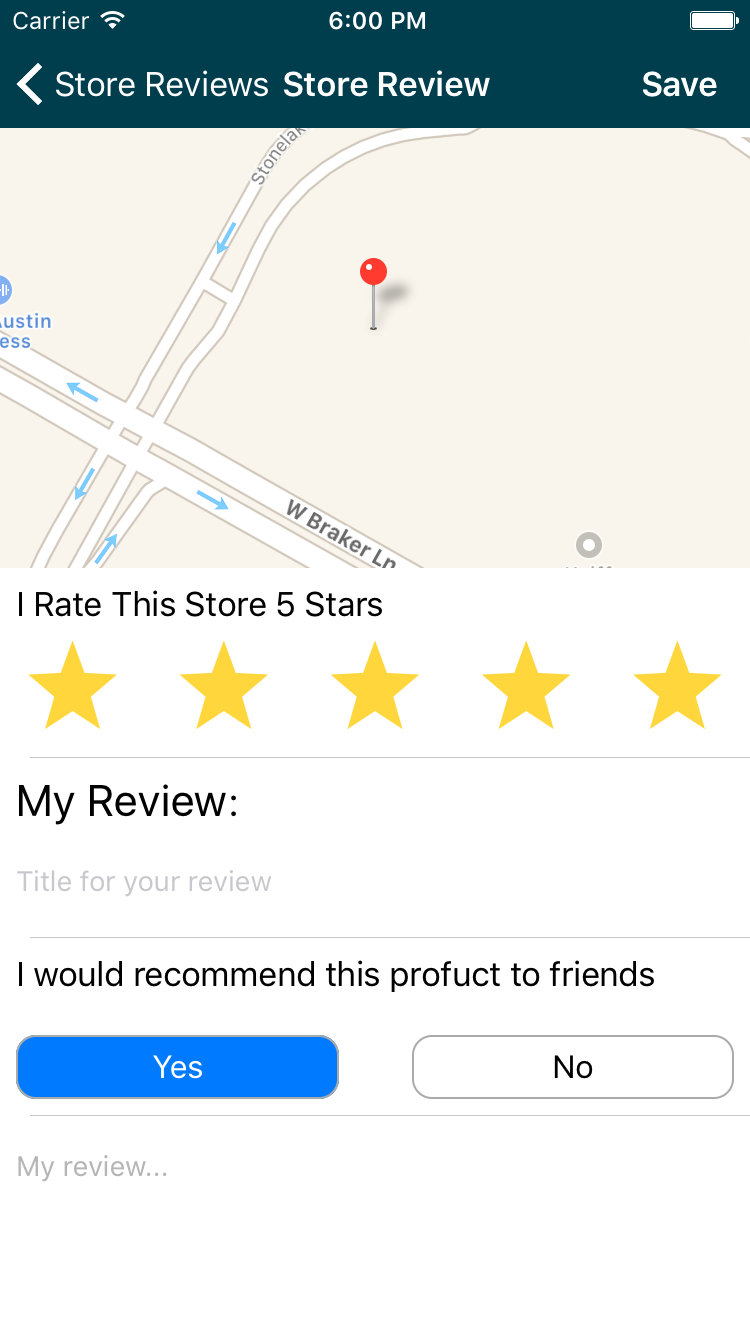
let reviewSubmission = BVStoreReviewSubmission(reviewTitle: "Title",
reviewText: "review text goes here....",
rating: 5,
productId: storeId
reviewSubmission.action = BVSubmissionAction.Preview // Change to .Submit to write for real
let userId = "123abc"
let userNickName = "userNick"
let userEmail = "[email protected]"
reviewSubmission.user = userId
reviewSubmission.userNickname = userNickName
reviewSubmission.userEmail = userEmail
reviewSubmission.isRecommended = true
reviewSubmission.agreedToTermsAndConditions = true
reviewSubmission.addPhoto(photo, withPhotoCaption: nil)
reviewSubmission.submit({ (response) in
// Successful submission!
}) { (errors) in
// Error alert your user something went wrong and try again.
}
BVStoreReviewSubmission *reviewSubmit = [[BVStoreReviewSubmission alloc] initWithReviewTitle:@"Review Title" reviewText:@"review text...." rating:5 storeId:@"yourStoreId"];
reviewSubmit.action = BVActionPreview; // change to submit for live submissions!
// Add your other required parameters here...
[reviewSubmit submit:^(BVReviewSubmissionResponse * _Nonnull response) {
// Success!
} failure:^(NSArray * _Nonnull errors) {
// Failure :(
}];
Updated over 1 year ago