Introduction
This tutorial explains how to use OAuth2 with the Bazaarvoice Response API using a three-legged workflow, which requires a Bazaarvoice Portal user to supply credentials during the process.
Bazaarvoice has implemented 3-legged OAuth2, an open standard for access delegation. This style of OAuth is referred to as “3-legged” because it consists of three roles:
- The Client Application: This is an application that would like to access data or interact with a Bazaarvoice service on behalf of a user.
- The OAuth2 API: A Bazaarvoice service that implements the OAuth2 standard and intermediates between the User and Client Application.
- The User: This is the person who is using the Client Application. They can grant or deny the Client Application access to their data.
3-legged OAuth2 offers certain advantages including:
- The User’s credentials are never exposed to the Client Application.
- The Client Application can be used by an arbitrary number of users.
- As a well-known open standard, OAuth2 is easier to implement than a custom solution.
OAuth2 Components
The following table summarizes several components that will be used when implementing OAuth2.
Component | Description |
---|---|
Authorization Code | A code that, when combined with the Client Secret, allows the Client Application to request an Access Token. This value is provided by the OAuth2 API. An Authorization Code may only be used once and has a lifespan of 60 seconds. |
Access Token | A token that, when combined with the Client Secret, allows the Client Application to access data or interact with a Bazaarvoice service on behalf of a user. The Access Token is provided by the OAuth2 API and has a lifespan of 60 minutes. |
Refresh Token | A token that, when combined with the Client Secret, can be used to request a new Access Token. Refresh tokens have unlimited lifetime as long as they are used at least every 7 days. |
Client Secret | A value used to verify the identity of the Client Application. This value will be provided by Bazaarvoice when the Client Application is registered. The Value should NOT be exposed to the public |
Client ID | A value used to identify the Client Application. This value will be provided by Bazaarvoice when the Client Application is registered. |
Redirect URI | This is a URL that identifies a resource implemented by the Client Application. The Authorization Service will send the User back to this location after the User logs into Bazaarvoice. This value must be provided to Bazaarvoice when the Client Application is registered. The Authentication Service will not redirect to unknown locations. |
Walkthrough
All requests to the OAuth2 API must use HTTPS.
The following sections describe the recommended method for implementing authentication for your application. The API calls you make to the OAuth2 API count towards your passkey's rate limit and quota, so correct implementation is highly recommended.
The Bazaarvoice OAuth2 integration can be divided into the following action:
- Authorization
- Description: The Client Application initiates a process in which the User can grant or deny access to the Client Application by logging in to the Bazaarvoice Portal through their browser. If access is granted, the Client Application will receive an
Authorization Code
from Bazaarvoice. - When to perform: When you don’t have a valid Access Token or valid Refresh Token.
- Description: The Client Application initiates a process in which the User can grant or deny access to the Client Application by logging in to the Bazaarvoice Portal through their browser. If access is granted, the Client Application will receive an
- Token exchange
- Description: Client Application submits the Client Secret and Client ID to Bazaarvoice. If they are valid, Bazaarvoice will return an Access Token that the Client Application can use when making requests to a Bazaarvoice Service.
- When to perform: When you don’t have a valid Access Token.
- Token refresh
- Description: When an Access Token is expired, or nearing expiration, a Client Application can request a new Access Token by submitting the Refresh Token to the OAuth2 API.
- When to perform: When you have a valid Refresh Token and the Access Token is expired or will expire very soon.
Each action is described in more detail below.
Authorization
Step 1: Request Login Page
To begin the authorization process, the Client Application should make a request to the OAuth2 API as depicted below. If you are testing this step during development, you can perform this step in a browser to automatically be redirected to the login page in the next step without having to extract the redirect location.
Request
GET https://[stg.]api.bazaarvoice.com/auth/v1/oauth2/auth?client_id={CLIENT_ID}&redirect_uri={REDIRECT_URI}&passkey={PASSKEY}
The value used for
{REDIRECT_URI}
must match exactly with the URL provided to Bazaarvoice during application registration.
Assuming the client_id
, passkey
and redirect_uri
are valid, the API will respond with a 303 redirect with a Location
header that passes along query strings from API request.
Response
HTTP/1.1 303 See Other
Connection keep-alive
Content-Length 0
Location https://portal.bazaarvoice.com/oauth2?client_id={CLIENT_ID}&redirect_uri={REDIRECT_URI}&client_name={CLIENT_DISPLAY_NAME}
...
Ellipsis (…) indicate that some headers have been omitted.
https://portal.bazaarvoice.com/oauth2
is not a part of the public facing Bazaarvoice OAuth2 API. Client Applications should not make requests to it directly.
Step 2: Access page
Next, the application must redirect the User’s browser to the URL identified in the Location header of the previous response:
The following request and response occur on the User's browser.
Request
GET https://portal.bazaarvoice.com/oauth2?client_id={CLIENT_ID}&redirect_uri={REDIRECT_URI}&client_name={CLIENT_DISPLAY_NAME}
https://portal.bazaarvoice.com/oauth2
is not a part of the public facing Bazaarvoice OAuth2 API. Client Applications should not make requests to it directly.
The Users will see the Bazaarvoice Portal access screen similar to the image below
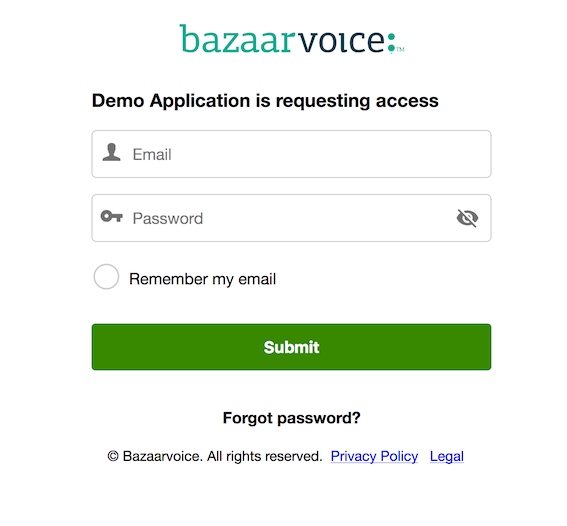
The User will be presented with the option to grant the Client Application access on behalf of the User.
Step 3: Back to the Client Application
If the User grants access to the Client Application by logging in, then the Bazaarvoice Portal will redirect the user's browser to the URL identified in the redirect_uri
parameter, sending the User back to the Client Application along with an Authorization Code.
The following request and response occur on the User's browser.
Request
GET {REDIRECT_URI}?code={AUTHORIZATION_CODE}
Response
The User will be redirected to your application's redirect URI. The Client Application should extract the Authorization Code from the URL to request an Access Token within 60 seconds, as described in the next step, or the Authorization Code will expire. If Token exchange is successful, then the Client Application should respond with the appropriate application state. If Token exchange is unsuccessful, then consider loading an error page that invites the User to re-attempt giving access.
Now that the Client Application has a valid Authorization Code it can perform the Token exchange.
Token Exchange
Step 1: Requesting an Access Token with an Authorization Code
The Client Application should perform a token exchange when it receives a request to the Redirect URI that includes the code parameter.
The token exchange is accomplished by submitting the Authorization Code, along with several other values, to the OAuth2 API, as depicted below:
This request should be done on the server and should use HTTPS.
Request :
POST https://[stg.]api.bazaarvoice.com/auth/v1/oauth2/token?passkey={API_PASSKEY}
Content-Type: application/x-www-form-urlencoded
…
code={AUTHORIZATION_CODE}&grant_type=authorization_code&client_id={CLIENT_ID}&redirect_uri={REDIRECT_URI}&client_secret={CLIENT_SECRET}
Ellipsis (…) in the example above indicate your application may generate other headers.
If successful, the OAuth2 API will respond with the following Access Token data:
Response
{
"access_token": "{ACCESS_TOKEN}",
"token_type": "Bearer",
"expires_at": {TIME_STAMP},
"scope": "offline_access",
"refresh_token": "{REFRESH_TOKEN}"
}
The Access Token can now be used to authenticate requests to other Bazaarvoice services.
Step 2: Persist the token data
The relationship between the token response object and the corresponding user should be persisted in the User’s browser session, for example with a cookie, so that it can be used for subsequent requests for the same user.
The Client Application should also store the entire token response object. Exactly how this is accomplished is up to the Client Application developer.
Don't expose the token response object to the public. It should be kept private and secure at all times.
Next
Now the Client Application can use the Access Token to make secure requests to Bazaarvoice on behalf of the User.
Before each request, verify that the Access Token is still valid and will not expire in the near future. If the Access Token is expired or will expire soon, then perform the Token refresh described below to get a new Access Token.
Token refresh
Step 1: Requesting an Access Token with a Refresh Token
To request a new Access Token, the Client Application should submit a Refresh Token to the OAuth2 API. If the Refresh Token is valid, then the OAuth2 API will respond with new Access Token data.
The following request demonstrates how to request new Access Token data:
This request should be done on the server and should use HTTPS.
POST https://[stg.]api.bazaarvoice.com/auth/v1/oauth2/token?passkey={API_PASSKEY}
Content-Type: application/x-www-form-urlencoded
…
refresh_token={REFRESH_TOKEN}&grant_type=refresh_token&client_id={CLIENT_ID}&redirect_uri={REDIRECT_URI}&client_secret={CLIENT_SECRET}
Ellipsis (…) in the example above indicate your application may generate other headers.
Response
If successful, the OAuth2 API will respond with the following token response object:
{
"access_token": "{ACCESS_TOKEN}",
"token_type": "Bearer",
"expires_at": {TIME_STAMP},
"scope": "offline_access",
"refresh_token": "{REFRESH_TOKEN}"
}
The Access Token can now be used to authenticate requests with the Bazaarvoice Response API.
Step 2: Persist the token data
The relationship between the token response object and the corresponding User should be persisted in the User’s browser session, for example with a cookie, so that it can be used for subsequent requests for the same user.
The Client Application should also store the entire token response object. Exactly how this is accomplished is up to the Client Application developer.
Don't expose the token response object to the public. It should be kept private and secure at all times.
Next
Now the Client Application can use the Access Token to make secure requests to Bazaarvoice on behalf of the User.
Before each request, verify that the Access Token is still valid and will not expire in the near future. If the Access Token is expired or will expire soon, then perform the Token refresh described above to get a new Access Token.